Neovim: From simple texteditor to IDE like
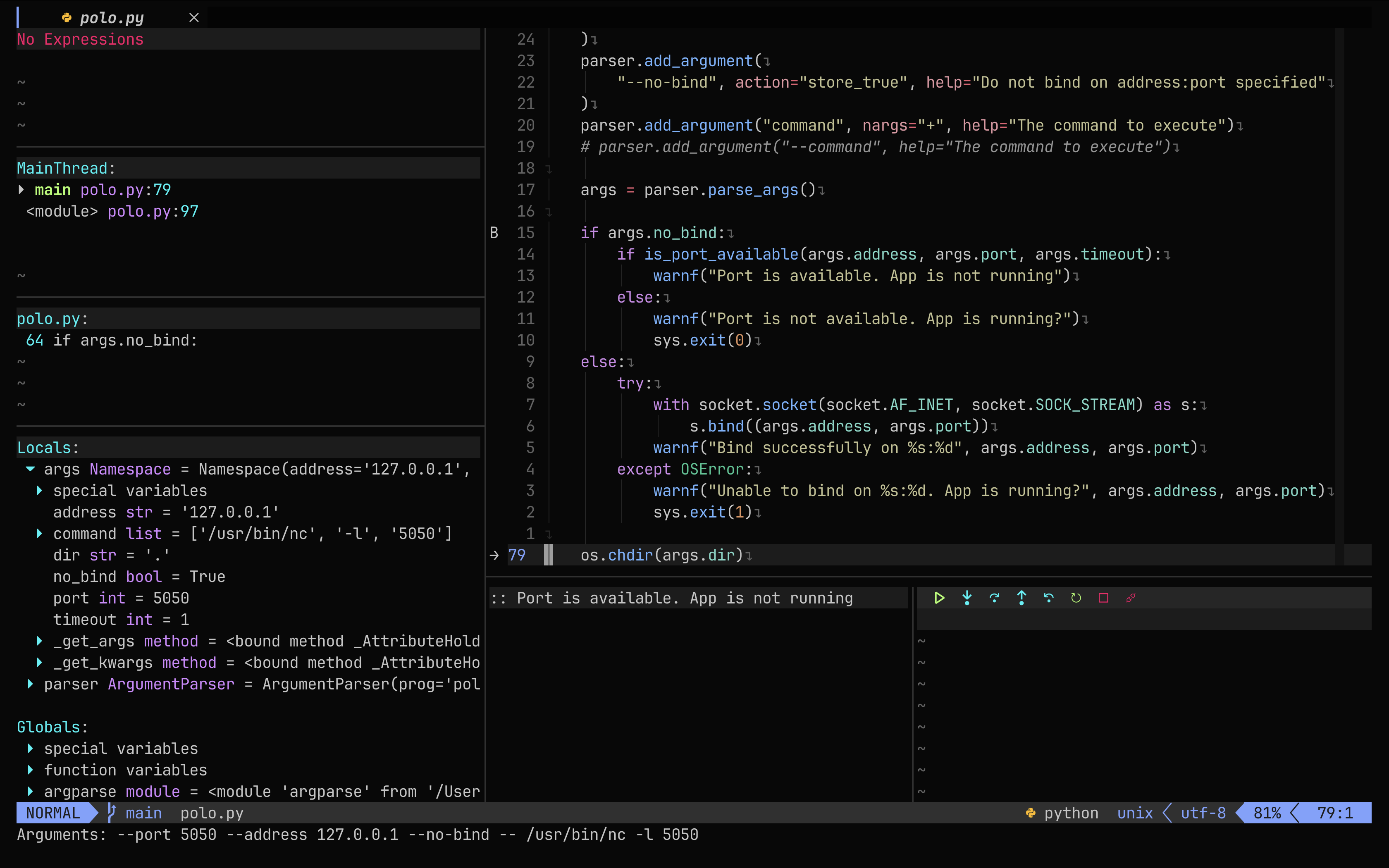
Hi, it’s Kn.
Neovim comes with cool new improvements like Lua remote plugin host, built-in LSP client (yes!), and Treesitter syntax engine I found there are already a bunch of great plugins that leverage those features. I tried them, and already love them! Also Since my current laptop is quite old ( a Macbook with core i5 gen 7th processor and 8gb ram ), Sublime Text or Vscode is too “hard” for him to handle.
I’d like to introduce my latest setup with Neovim 0.9 and modern plugins. Here is a quick summary of my set up:
- lazy.nvim - is a modern plugin manager for Neovim
- LSP-zero - A starting point to setup some lsp related features in neovim.
- mason - Easily install and manage LSP servers, DAP servers, linters, and formatters
- mason-lspconfig - Extension to mason.nvim that makes it easier to use lspconfig with mason.nvim.
- nvim-cmp - A completion plugin for neovim coded in Lua.
- mason-null-Ls - bridges mason.nvim with the null-ls plugin, making it easier to use both plugins together.
- nvim-treesitter - Treesitter configurations and abstraction layer for Neovim
- telescope.nvim - A highly extendable fuzzy finder over lists
- lualine.nvim - A blazing fast and easy to configure neovim statusline plugin written in pure lua
- nvim-tree.lua - A file explorer tree for neovim written in lua
- Indent-blank-line - Indent guides for Neovim
- gitsigns - Git integration for buffers
- nvim-dap-ui, nvim-dap - Debug Adapter Protocol client implementation for Neovim
- autopairs - autopairs for neovim
- undotree - The undo history visualizer for VIM
And here is my dotfiles repository:
https://github.com/huyhoang8398/dotfiles
Prerequisites — iTerm2 and Patched Nerd Font
iTerm2 is a fast terminal emulator for macOS. Install one of Nerd Fonts for displaying fancy glyphs on your terminal. My current choice is JetBrainsMono Nerdfont. And use it on your terminal app. For example, on iTerm2:
My color theme is Moonfly, which is a true color Moonfly theme. The Moonfly theme for iTerm2 is in my dotfile repository here with a bit color customization.
Type | Category | Value | Color |
---|---|---|---|
Background | Background | #080808 | |
Foreground | Foreground | #bdbdbd | |
Bold | Bold | #eeeeee | |
Cursor | Cursor | #9e9e9e | |
Cursor Text | Cursor Text | #080808 | |
Selection | Selection | #b2ceee | |
Selection Text | Selection Text | #080808 | |
Color 1 | Black (normal) | #323437 | |
Color 2 | Red (normal) | #ff5454 | |
Color 3 | Green (normal) | #8cc85f | |
Color 4 | Yellow (normal) | #e3c78a | |
Color 5 | Blue (normal) | #80a0ff | |
Color 6 | Purple (normal) | #cf87e8 | |
Color 7 | Cyan (normal) | #79dac8 | |
Color 8 | White (normal) | #c6c6c6 | |
Color 9 | Black (bright) | #949494 | |
Color 10 | Red (bright) | #ff5189 | |
Color 11 | Green (bright) | #36c692 | |
Color 12 | Yellow (bright) | #c2c292 | |
Color 13 | Blue (bright) | #74b2ff | |
Color 14 | Purple (bright) | #ae81ff | |
Color 15 | Cyan (bright) | #85dc85 | |
Color 16 | White (bright) | #e4e4e4 |
Install Neovim
Directory structure
Neovim conforms XDG Base Directory structure
. Here is my config file structure:
.
├── init.lua | Neovim initialization file
├── after/ | Standard auto-loading 'after' base directory
│ └── plugin/ | Auto-loading 'plugin' configs
├── ftdetect/ | Detect filetype
└── lua/ | Lua base directory
├── config/ | Plugin configs that ARE lazy-loaded via explicit 'require'
└── custom/ | plugin-manager, mappings and options configs
Install plugin manager: Lazy.nvim
Lazy.nvim comes with lots of features that I found useful sunch as:
- 📦 Manage all your Neovim plugins with a powerful UI
- 🚀 Fast startup times thanks to automatic caching and bytecode compilation of Lua modules
- 💾 Partial clones instead of shallow clones
- 🔌 Automatic lazy-loading of Lua modules and lazy-loading on events, commands, filetypes, and key mappings
- ⏳ Automatically install missing plugins before starting up Neovim, allowing you to start using it right away
- 💪 Async execution for improved performance
- 🛠️ No need to manually compile plugins
- 🧪 Correct sequencing of dependencies
- 📁 Configurable in multiple files
- 📚 Generates helptags of the headings in README.md files for plugins that don’t have vimdocs
- 💻 Dev options and patterns for using local plugins
- 📊 Profiling tools to optimize performance
- 🔒 Lockfile lazy-lock.json to keep track of installed plugins
- 🔎 Automatically check for updates
- 📋 Commit, branch, tag, version, and full Semver support
- 📈 Statusline component to see the number of pending updates
- 🎨 Automatically lazy-loads colorschemes
Setup
Firstly, create a lazy.lua
file in ~/.config/nvim/lua/custom/
with this content
You then can add the following Lua code to your lazy.lua
to bootstrap lazy.nvim
:
Next step is to add lazy.nvim below the code added in the prior step in lazy.lua
:
plugins
: this is all the plugin that you want to install and should be a table or a string
table
: a list with your Plugin Specstring
: a Lua module name that contains your Plugin Spec.
opts
: this should be lazy nvim options itself and should be a table.
Example Options
Example Plugin Install
In this Blog, I will cover only some essenstial plugins, the rest you could see in my dotfiles
link above
Colorscheme
Im using Moonfly - a dark charcoal theme with some options:
It supports treesitter and other plugins very well and Also the maintainer is really friendly (We talk alots in Discord)
Status line: Lualine
nvim-lualine/lualine provides a flexible way to configure statusline
LSP Zero - LSP Lifesaver
The purpose of this plugin is to bundle all the “boilerplate code” necessary to have nvim-cmp
(a popular autocompletion plugin) and nvim-lspconfig
working together. And if you opt in, it can use mason.nvim
to let you install language servers from inside neovim.
It used to be very difficult to setup language server, autocompletion, etc with CoC or similar, but now Neovim has a built-in LSP support. You can easily configure it by using neovim/nvim-lspconfig
and Mason
.
For me I found that LSP zero is lifesaver but you could also consider you might not need lsp-zero.
LSP setup
My above template will cover all requirement for LSP, Autocompletion and Snippet
Language servers are configured and initialized using nvim-lspconfig
.
Example Config
Install a language server
Let’s try to use the language server for lua.
Open your init.lua
and execute the command :LspInstall
. Now mason.nvim
will suggest a language server. Neovim should show a message like this.
Please select which server you want to install for filetype "lua":
1: lua_ls
Type number and <Enter> or click with the mouse (q or empty cancels):
Choose 1 for lua_ls
, then press enter. A floating window will show up. When the server is done installing, a message should appear.
At the moment the language server can’t start automatically, restart Neovim so the language server can be configured properly. Once the server starts you’ll notice warning signs in the global variable vim, that means everything is well and good.
For automatically installing any language server, you could create a file in `after/plugin/mason-lspconfig.lua
I mainly use Bash, Python and Go so it will will automtically install these languague server
If you want to manully install this, try to run :Mason
, a popup screen will show like this and you could chose whatever language server you want to install
Auto-completion: cmp
To get LSP-aware auto-completion feature with fancy pictograms, I use the following plugins:
- L3MON4D3/LuaSnip - Snippet engine
- hrsh7th/cmp-nvim-lsp - nvim-cmp source for neovim’s built-in LSP
- hrsh7th/cmp-buffer - nvim-cmp source for buffer words
- hrsh7th/nvim-cmp - A completion engine plugin for neovim
- hrsh7th/cmp-path - Path completion
- hrsh7th/cmp-nvim-lsp-signature-help - Signature help
Configure it like so:
Syntax highlightings: Treesitter
Treesitter is a popular language parser for syntax highlightings. First, install it:
Install nvim-treesitter/nvim-treesitter
with Lazy and configure it like so:
Fuzz finder: Telescope
telescope.nvim
provides an interactive fuzzy finder over lists, built on top of the latest Neovim features. I also use telescope-file-browser.nvim as a filer.
It’s so useful because you can search files while viewing the content of the files without actually opening them. It supports various sources like Vim, files, Git, LSP, and Treesitter. Check out the showcase of Telescope.
Install kyazdani42/nvim-web-devicons to get file icons on Telescope, statusline, and other supported plugins.
The configuration would look like so:
keymaps
Code formatter: null-ls
I use null-ls for code formatting and extra diagnostics The config example as following:
Git markers: gitsigns
lewis6991/gitsigns.nvim
provides git decorations for current buffers. It helps you know which lines are currently changed. It works out of the box.
I made some configuration and hotkey to suite my usage
Undotree - my favorite extension
The undo history visualizer for VIM Install it with
should work out of box
Debugging
Im currently using mfussenegger/nvim-dap
for debugging, thanks to Microsoft Debug Adapter Protocol
with some keybind:
Finally, to combime all of this, you could add in your root init.lua
file:
...
That’s pretty much it! I hope it’s helpful for improving your Neovim environment.